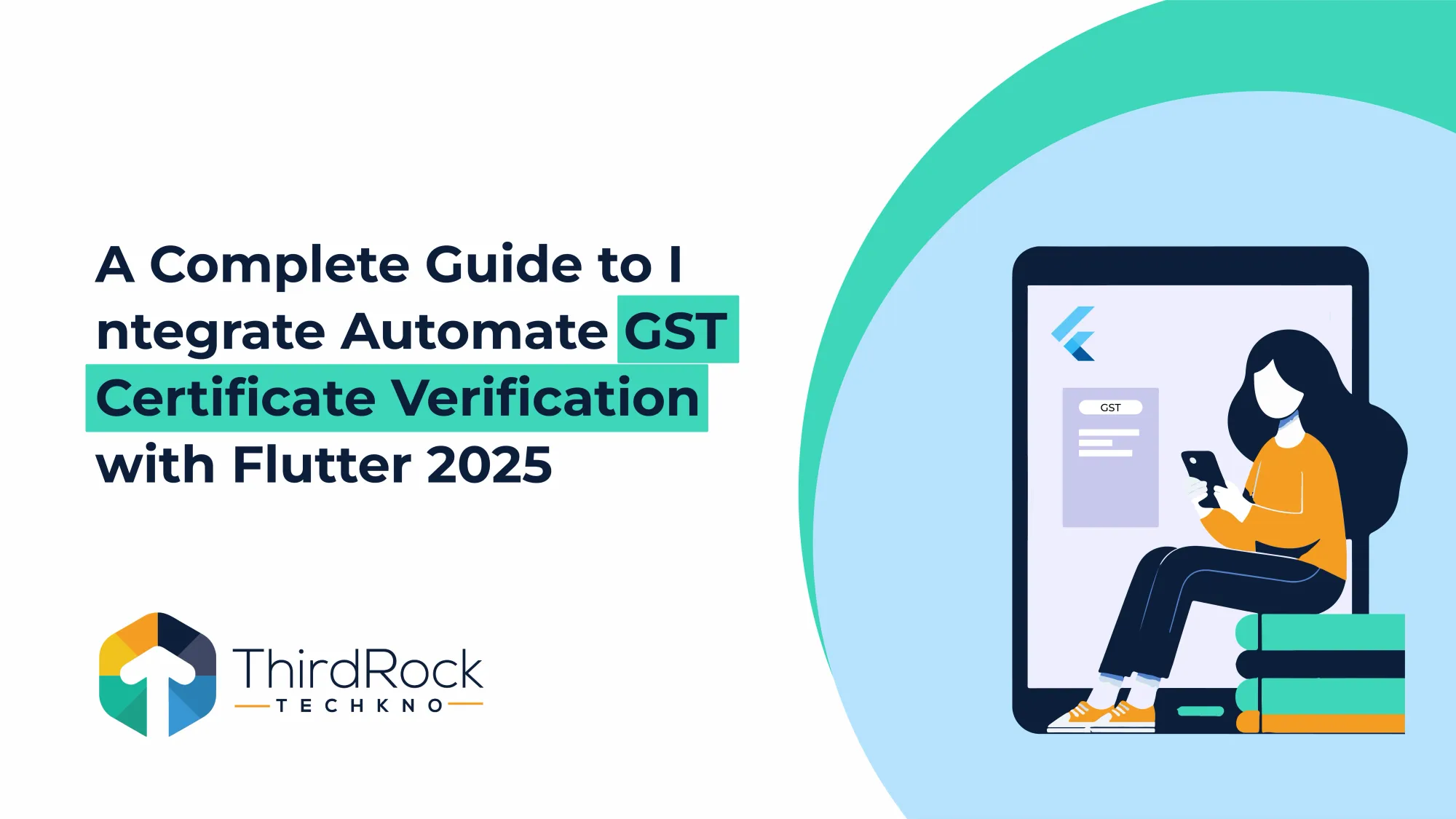
Table of Contents
Importance of Automated GST Certification Verification Using OCR
Step 1: Set Up a Flutter Project
Step 2: Add Required Dependencies
Step 3: Create a New API Service Class
In this guide, we will walk through the steps to implement automatic GST certificate verification in a Flutter app using the Idfy API, making it possible for businesses to verify the authenticity of GST certificates using OCR technology.
Importance of Automated GST Certification Verification Using OCR
Verifying GST certificates through OCR is important for businesses and financial institutions to validate GST certificates without manual intervention. Here are a few key reasons why GST certificate verification is essential:
- Operational Efficiency:- Manually verifying GST certificates can be time-consuming and error-prone. Automated systems can perform verification in real-time, speeding up the workflow and improving accuracy.
- Preventing Fraud:- Automated verification reduces the risk of using fake or invalid GST certificates for business transactions, safeguarding companies from potential tax liabilities.
- Regulatory Compliance: Many industries require valid GST certificates for partnerships, billing, or claiming tax credits. Automation ensures that these certificates are verified quickly and meet regulatory standards.
- Customer Trust: For businesses, having a robust GST verification system improves trust among partners and clients by ensuring accurate tax compliance and valid documentation.
We will use the following APIs from Idfy:
- POST API to extract GST certificate data from an image.
- GET API to retrieve the verification details using the request ID.
Prerequisites
- Flutter Environment: You need a working Flutter setup on your machine.
- Idfy API Access: Sign up for an account on Idfy and get your API key and account ID
- Image Picker Package: Add the image_picker package to your pubspec.yaml file to allow users to select and upload images.
Step 1: Set Up a Flutter Project
First, let's create a new Flutter project for GST certificate verification.
flutter create gst_verification_app
cd gst_verification_app
Open the project in your preferred IDE (VS Code, Android Studio, etc.)
Step 2: Add Required Dependencies
To make HTTP requests, we'll add the http package to the pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
http: ^0.13.3
image_picker: ^0.8.4+2Run the command to install the dependencies:flutter pub get
Step 3: Create a New API Service Class
Create a new file called gst_verification_service.dart in the lib folder of your Flutter project. This class will handle all the API request.
lib/gst_verification_service.dart:
import 'dart:convert';
import 'dart:io';
import 'package:http/http.dart' as http;
class GstVerificationService {
final String apiUrl = 'https://eve.idfy.com/v3/tasks/async/extract/ind_gst_certificate';
final String fetchUrl = 'https://eve.idfy.com/v3/tasks';
final String accountId = 'your_account_id'; // Replace with your account ID
final String apiKey = 'your_api_key'; // Replace with your API key
// Function to upload GST certificate and get the request ID
Future<String?> uploadGstCertificate(File imageFile) async {
try {
final bytes = imageFile.readAsBytesSync();
final String base64Image = base64Encode(bytes);
final response = await http.post(
Uri.parse(apiUrl),
headers: {
'account-id': accountId,
'api-key': apiKey,
'Content-Type': 'application/json',
},
body: jsonEncode({
'task_id': 'your_task_id',
'group_id': 'your_group_id',
'data': {
'document1': base64Image,
},
}),
);
if (response.statusCode == 200) {
final responseData = jsonDecode(response.body);
return responseData['request_id'];
} else {
print('Failed to upload GST certificate: ${response.body}');
return null;
}
} catch (e) {
print('Error uploading GST certificate: $e');
return null;
}
}
// Function to fetch GST details using the request ID
Future<Map<String, dynamic>?> fetchGstDetails(String requestId) async {
try {
final response = await http.get(
Uri.parse('$fetchUrl?request_id=$requestId'),
headers: {
'account-id': accountId,
'api-key': apiKey,
'Content-Type': 'application/json',
},
);
if (response.statusCode == 200) {
final responseData = jsonDecode(response.body);
return responseData['result']['extraction_output'];
} else {
print('Failed to fetch GST details: ${response.body}');
return null;
}
} catch (e) {
print('Error fetching GST details: $e');
return null;
}
}
}
Step 4: Update the Flutter UI to Use the Services
Now that we have the API integration logic in a service class, we will update the GstVerificationScreen in lib/main.dart to use the GstVerificationService.
lib/main.dart:
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:image_picker/image_picker.dart';
import 'gst_verification_service.dart';
void main() => runApp(GstVerificationApp());
class GstVerificationApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'GST Certificate Verification',
home: GstVerificationScreen(),
);
}
}
class GstVerificationScreen extends StatefulWidget {
@override
_GstVerificationScreenState createState() => _GstVerificationScreenState();
}
class _GstVerificationScreenState extends State<GstVerificationScreen> {
File? _image;
final picker = ImagePicker();
String? _gstDetails;
final GstVerificationService _gstVerificationService = GstVerificationService(); // Instantiate the service
Future<void> _pickImage() async {
final pickedFile = await picker.pickImage(source: ImageSource.gallery);
if (pickedFile != null) {
setState(() {
_image = File(pickedFile.path);
});
_uploadGstCertificate(_image!);
}
}
Future<void> _uploadGstCertificate(File imageFile) async {
final requestId = await _gstVerificationService.uploadGstCertificate(imageFile);
if (requestId != null) {
_fetchGstDetails(requestId);
}
}
Future<void> _fetchGstDetails(String requestId) async {
final details = await _gstVerificationService.fetchGstDetails(requestId);
setState(() {
_gstDetails = details != null ? details.toString() : 'Failed to retrieve GST details';
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('GST Certificate Verification'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
children: [
_image == null
? Text('No image selected.')
: Image.file(_image!, height: 200),
SizedBox(height: 16),
ElevatedButton(
onPressed: _pickImage,
child: Text('Upload GST Certificate'),
),
SizedBox(height: 16),
_gstDetails == null
? Text('GST details will appear here.')
: Text('GST Details: $_gstDetails'),
],
),
),
);
}
}
Explanation
- GstVerificationService Class:
- This class contains two methods: one for uploading the GST certificate (uploadGstCertificate) and another for fetching GST details (fetchGstDetails).
- The uploadGstCertificate method takes the file, converts it to Base64, and sends it to the Idfy API to initiate the OCR task.
- The fetchGstDetails method retrieves the extracted GST details using the request ID.
2. Main Dart File:
- We refactored the code to use the GstVerificationService class.
- When the user uploads an image, the app calls the uploadGstCertificate method, which then calls fetchGstDetails to retrieve the GST information.
Step 5: Run the App
Test the app by selecting a GST certificate image. The app will upload the image, retrieve the extracted details, and display them on the screen.
Conclusion
By using the Idfy API, we have successfully implemented automated GST certificate verification in a Flutter application. By integrating these APIs, you can streamline GST verification in real-time and enhance the user experience by allowing users to upload a GST certificate, process it through OCR and retrieve important details such as the legal name, trade name, and registration type.
If you are looking for Flutter development we can build you built flutter app faster and ever. Visit us today.
API Reference: Official Documentation.
FAQs
- What is automated GST certificate verification?
Automated GST certificate verification is a process that uses OCR (Optical Character Recognition) technology to scan and validate GST certificates without manual intervention. This helps businesses ensure compliance, reduce fraud, and save time by automatically verifying the authenticity of certificates. - Why is automated GST certificate verification important for businesses?
Automating GST certificate verification is crucial for improving operational efficiency, preventing fraud, ensuring regulatory compliance, and building customer trust. By eliminating manual processes, businesses can speed up verification, reduce errors, and avoid tax liabilities due to fake or invalid GST certificates. - What is the role of the Idfy API in GST certificate verification?
The Idfy API facilitates the automation of GST certificate verification. It offers a POST API to extract GST certificate data using OCR technology and a GET API to retrieve verification details through a request ID. These APIs streamline the process by providing real-time results and eliminating manual checks. - How does OCR help in GST certificate verification?
OCR (Optical Character Recognition) scans the GST certificate, extracts text data like legal name, trade name, and registration type, and allows the system to verify the details automatically. This helps to minimize manual effort and errors in verifying the GST certificate. - Is the GST verification process secure?
Yes, the Idfy API uses secure endpoints and headers to ensure that the data transmission, including certificate uploads and retrievals, is handled securely. Your API key and account ID are used to authenticate the request.