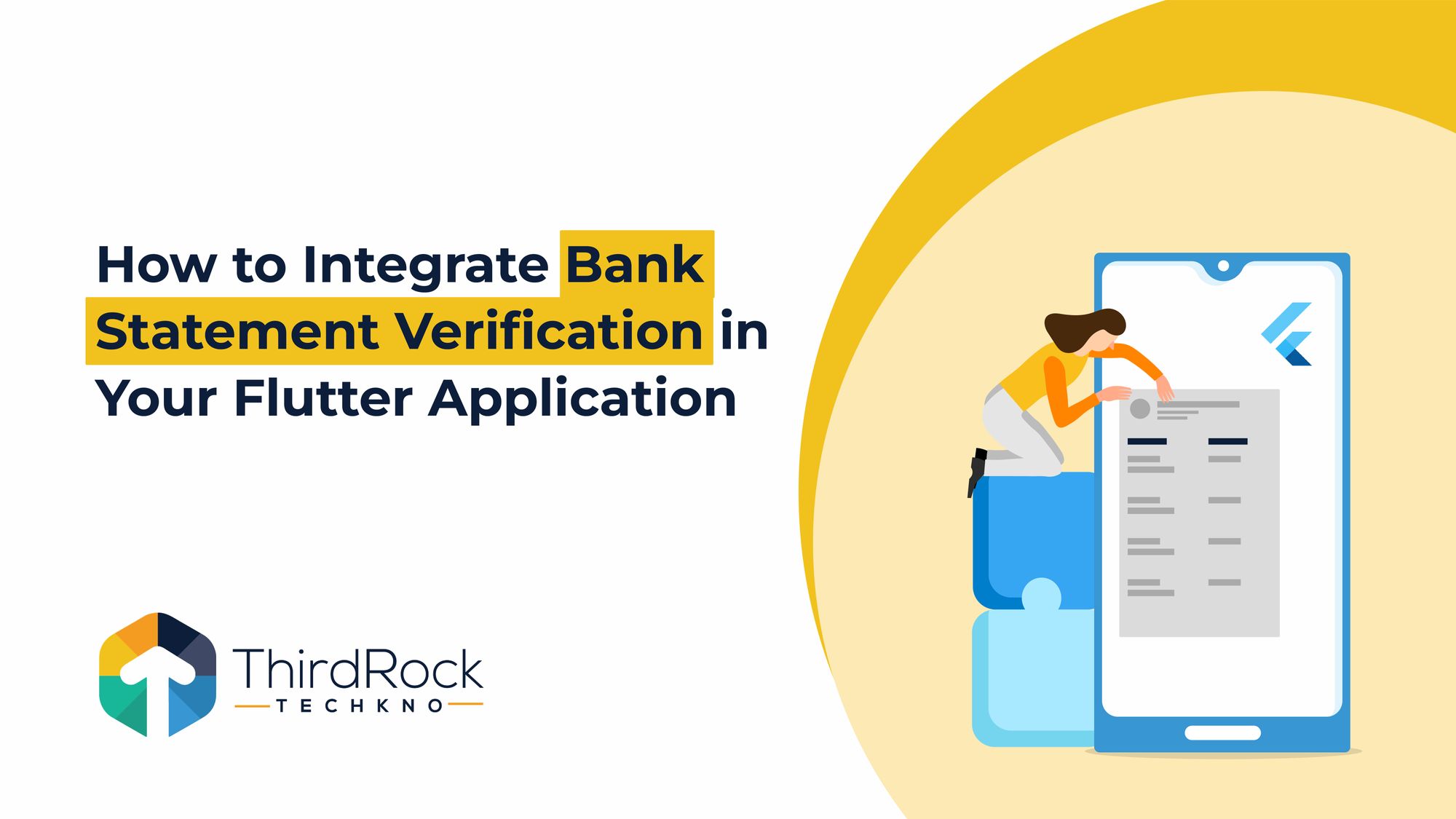
This guide will walk you through integrating bank statement verification into your Flutter app using IDFY’s Bank Statement API. We’ll focus on uploading a bank statement PDF and triggering verification.
Table of Contents
Use Cases for Bank Statement Verification
Bank statement verification is important for financial insititutions in making credit or loan- related decisions. Here are some common use cases
Salary Verification: Lenders can determine if the applicant's salary meets the criteria for loans.
Financial Health: By analyzing inflow, outflow, and balances, banks can assess the financial stability of individuals.
Risk Detection: The API can flag suspicious transactions and provide insights into potential fraudulent behavior.
Credit Decision: A complete view of the applicant's bank statement can influence loan approvals and credit limits.
Watch How Bank Statement Verification works
Prerequisites
Before starting, ensure you have:
Flutter SDK installed.
IDFY API Key.
Flutter dependencies:
http: for making API requests.
file_picker: for selecting and uploading a PDF file.
Update your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
http: ^0.13.4
file_picker: ^4.5.1
path_provider: ^2.0.11
RUN
Flutter pub get
Step 1: Set Up the Flutter Project
Create a new Flutter project or use an existing one
Install the required packages (http and file_picker) by adding them to your pubspec.yaml file as mentioned above.
Step 2: Create the Services Class to Handle API Calls
Now, create a service class to handle the API request for uploading the bank statement and checking the verification status.
Step 3: Create the UI to Upload Bank Statement PDF
import 'dart:convert';
import 'package:http/http.dart' as http;
class BankStatementService {
static const String apiUrl = 'https://eve.idfy.com/v3/tasks/async/verify_with_source/ind_bank_statement';
static const String apiKey = 'YOUR_API_KEY';
// Method to upload bank statement for verification
Future<String?> uploadBankStatement(String bankName, String accountType, String fileUrl, {String? password}) async {
final requestBody = {
"task_id": "74f4c926-250c-43ca-9c53-453e87ceacd1",
"group_id": "8e16424a-58fc-4ba4-ab20-5bc8e7c3c41e",
"data": {
"bank_statement": fileUrl,
"bank_name": bankName,
"account_type": accountType,
"password": password ?? ""
}
};
final response = await http.post(
Uri.parse(apiUrl),
headers: {
'api-key': apiKey,
'Content-Type': 'application/json',
},
body: jsonEncode(requestBody),
);
if (response.statusCode == 200) {
final data = jsonDecode(response.body);
return data['request_id']; // Returns the request ID for further status checks.
} else {
return null;
}
}
// Method to get verification result
Future<Map<String, dynamic>?> getVerificationResult(String requestId) async {
final response = await http.get(
Uri.parse('https://eve.idfy.com/v3/tasks?request_id=$requestId'),
headers: {
'api-key': apiKey,
},
);
if (response.statusCode == 200) {
return jsonDecode(response.body); // Returns the verification result.
} else {
return null;
}
}
}
Next, we’ll build a UI to allow users to upload a PDF bank statement and submit it for verification.
Step 4: Getting the Verification Result
import 'package:flutter/material.dart';
import 'package:file_picker/file_picker.dart';
import 'package:your_project/services/bank_statement_service.dart';
import 'dart:io';
class BankStatementScreen extends StatefulWidget {
@override
_BankStatementScreenState createState() => _BankStatementScreenState();
}
class _BankStatementScreenState extends State<BankStatementScreen> {
String? _bankName;
String? _accountType;
File? _pdfFile;
bool _isLoading = false;
final BankStatementService _service = BankStatementService();
// Pick PDF file
Future<void> _pickPDF() async {
FilePickerResult? result = await FilePicker.platform.pickFiles(
type: FileType.custom,
allowedExtensions: ['pdf'],
);
if (result != null) {
setState(() {
_pdfFile = File(result.files.single.path!);
});
}
}
// Handle the verification process
Future<void> _verifyBankStatement() async {
if (_bankName != null && _accountType != null && _pdfFile != null) {
setState(() {
_isLoading = true;
});
// You'd typically upload the file to your server or cloud storage to get a URL
String pdfUrl = "http://www.africau.edu/images/default/sample.pdf"; // Simulated PDF URL for this example
String? requestId = await _service.uploadBankStatement(
_bankName!,
_accountType!,
pdfUrl,
);
if (requestId != null) {
// Now, check the result of the verification
var result = await _service.getVerificationResult(requestId);
print("Verification Result: $result");
}
setState(() {
_isLoading = false;
});
} else {
// Handle error
print("All fields are required");
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Bank Statement Verification')),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
TextField(
decoration: InputDecoration(labelText: 'Bank Name'),
onChanged: (value) => _bankName = value,
),
TextField(
decoration: InputDecoration(labelText: 'Account Type (e.g. SAVING)'),
onChanged: (value) => _accountType = value,
),
SizedBox(height: 20),
Text(_pdfFile != null ? _pdfFile!.path : 'No file selected'),
ElevatedButton(
onPressed: _pickPDF,
child: Text('Pick Bank Statement PDF'),
),
SizedBox(height: 20),
_isLoading
? CircularProgressIndicator()
: ElevatedButton(
onPressed: _verifyBankStatement,
child: Text('Verify'),
),
],
),
),
);
}
}
Once the bank statement has been uploaded, you can fetch the result by calling the getVerificationResult method using the request ID.
Future<void> _verifyBankStatement() async {
if (_bankName != null && _accountType != null && _pdfFile != null) {
setState(() {
_isLoading = true;
});
String pdfUrl = "http://www.africau.edu/images/default/sample.pdf"; // Use actual file upload URL.
String? requestId = await _service.uploadBankStatement(
_bankName!,
_accountType!,
pdfUrl,
);
if (requestId != null) {
var result = await _service.getVerificationResult(requestId);
if (result != null) {
print("Verification Result: $result");
// Show result to the user or process the result further
}
}
setState(() {
_isLoading = false;
});
}
}
Conclusion
In this guide, we integrated bank statement verification in a Flutter app using IDFY’s Bank Statement API. We covered:
Uploading a PDF bank statement using a file picker.
Sending the file for verification via an API request
Retrieving the verification result using the request ID.
Official IDFY API
How to Navigate the Documentation
Go to the official IDFY documentation.
Select the "Verification Solutions" from the sidebar.
Select your country folder (e.g., India).
Open the "Async" folder to find the asynchronous API for bank statement verification.
Here, you'll find detailed information about the API, request formats, and responses required to successfully integrate the service into your application.
By following these steps, you can access the full API reference and learn more about customizing your Flutter integration for bank statement verification.