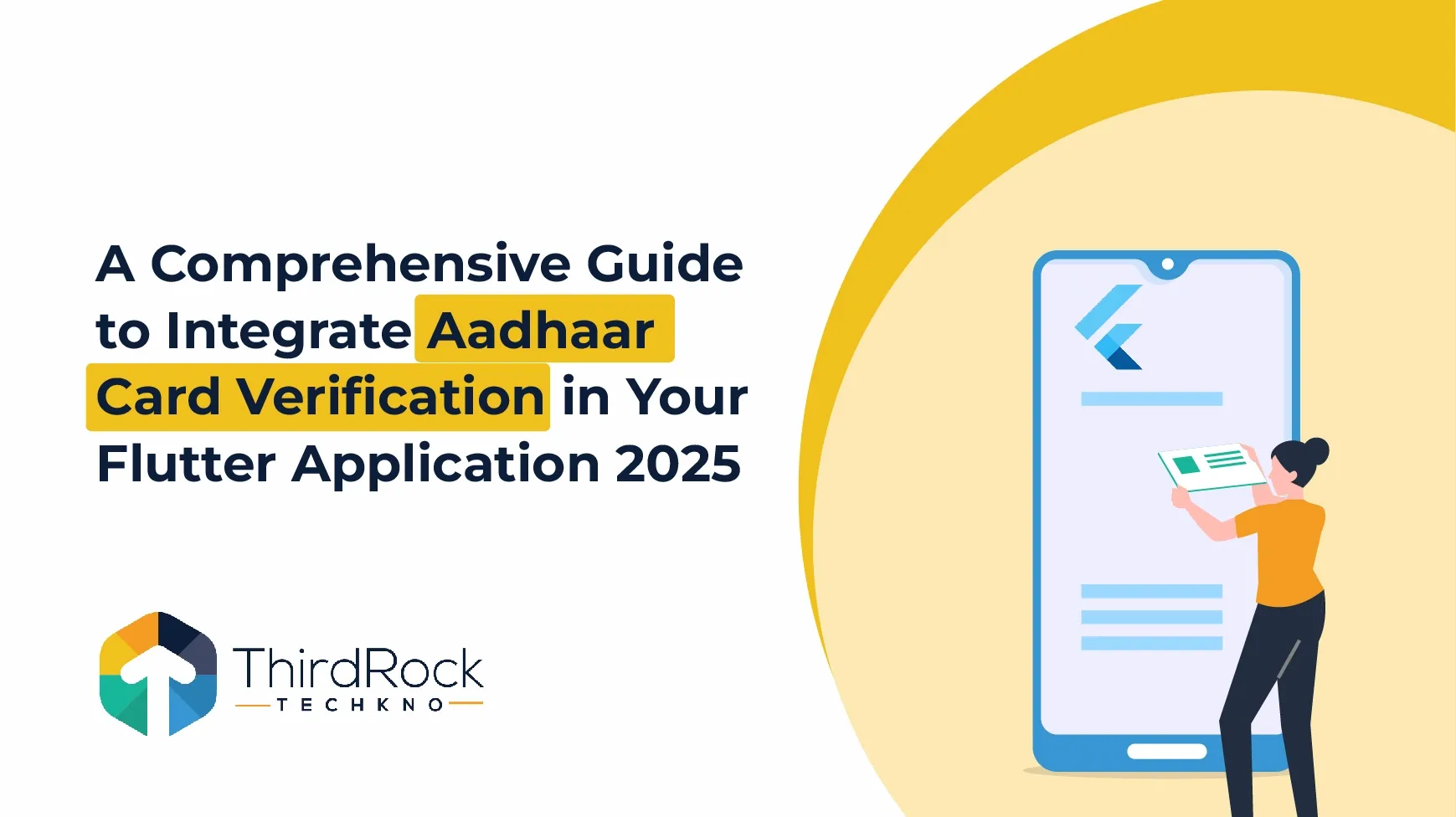
Using Setu's OKYC APIs, we will demonstrate how you can integrate Aadhaar Card verification into your Flutter application. We will set up the environment, make API calls, and handle the responses.
Table of Contents
How Aaddhar Verification Works
Before diving into the implementation, here’s a video that demonstrates how Aadhaar verification works, explaining the overall process:
Why Aadhaar Verification is Critical in Fintech Projects
Aadhaar card verification plays a crucial role in many financial and fintech projects. Here are a few key scenarios where Aadhaar verification is essential
KYC Compliance:- Fintech companies need to verify customer identities to comply with the Reserve Bank of India’s regulations. Aadhaar verification helps to streamline KYC processes quickly and efficiently.
Account Creation:- Digital banking apps and financial platforms often require Aadhaar verification as a part of their onboarding process to verify the authenticity of users.
Loan Disbursement and Insurance:- Aadhaar verification helps verify a user's identity before disbursing loans or approving insurance policies, ensuring the authenticity of the applicant.
Preventing Fraud:- Aadhaar verification reduces the risk of fraud by confirming that the person attempting to access services is who they claim to be.
Prerequisites
Flutter development environemnt set up on your machines.
A Setu account with access to their Aadhaar verification API.
API credentials (x-cilent-id, x-client-secret, x-product-instane-id) from setu.
Overview of Setu API
Create Aadhaar Verification Request: Initiates a verification request.
Get Aadhaar Verification Details: Fetches the status and details of the verification request.
Example Request and Response
Sandbox BaseURL:- ttps://dg-sandbox.setu.co
Production BaseURL: https://dg.setu.co
Create Aadhar Verification Request. Official Doc
CURL:
{
"redirectURL": "https://setu.co"
}
Response:
{
"id": "7097e53a-ba29-48a2-983d-878433b4f33e",
"url": "https://setuOKYCURL.co/foobar&sessionId=uuid",
"validUpto": "Wed, 23 Jun 2021 19:33:55 GMT",
"status": "incomplete"
}
Request Body:
2. Verify OKYC Request (Aadhar Car Verification). Official Doc
CURL:
curl --request POST \
--url https://dg-sandbox.setu.co/api/okyc/{requestId}/verify \
--header 'content-type: application/json' \
--header 'x-client-id: test-client' \
--header 'x-client-secret: YOUR_CLIENT_SECRET' \
--header 'x-product-instance-id: YOUR_X_PRODUCT_INSTANCE_ID' \
--data '{
"aadhaarNumber": "999999990019",
"captchaCode": "2GAD0"
}'
Request Body:
{
"aadhaarNumber": "999999990019",
"captchaCode": "2GAD0"
}
Response:
Official Setu Documentation
For more detailed information and additional API endpoints, refer to the Setu OKYC API documentation.
Integrating Aadhar Verification in Flutter
Step 1: Set Up Flutter Project
In case you don't already have one, create a new Flutter project:
flutter create aadhaar_verification_setu
cd aadhaar_verification_setu
Step 2: Add Dependencies
Open pubspec.yaml and add the http package for making API requests:
dependencies:
flutter:
sdk: flutter http: ^0.14.0
Run “flutter pub get” to install the dependencies.
Step 3: Creating an API Services Class to Handle API Calls
Create a new file lib/api_service.dart to handle the API requests.
import 'dart:convert';
import 'package:http/http.dart' as http;
class AadhaarVerificationService {
final String baseUrl = 'https://dg-sandbox.setu.co/api/okyc';
final String clientId = 'test-client';
final String clientSecret = 'YOUR_CLIENT_SECRET';
final String productInstanceId = 'YOUR_PRODUCT_INSTANCE_ID';
Future<Map<String, dynamic>> verifyAadhaarCard(
String requestId, String aadhaarNumber, String captchaCode) async {
final url = Uri.parse('$baseUrl/$requestId/verify');
final response = await http.post(
url,
headers: {
'Content-Type': 'application/json',
'x-client-id': clientId,
'x-client-secret': clientSecret,
'x-product-instance-id': productInstanceId,
},
body: jsonEncode({
'aadhaarNumber': aadhaarNumber,
'captchaCode': captchaCode,
}),
);
if (response.statusCode == 200) {
return jsonDecode(response.body);
} else {
throw Exception('Failed to verify Aadhaar card: ${response.reasonPhrase}');
}
}
}
Step 4: Creating the UI
Make a basic user interface (UI) in lib/main.dart that includes a TextField for the Aadhaar number and captcha code, as well as a Button to start the verification process.
import 'package:flutter/material.dart';
import 'api_service.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Aadhaar Verification',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: AadhaarVerificationScreen(),
);
}
}
class AadhaarVerificationScreen extends StatefulWidget {
@override
_AadhaarVerificationScreenState createState() => _AadhaarVerificationScreenState();
}
class _AadhaarVerificationScreenState extends State<AadhaarVerificationScreen> {
final TextEditingController _aadhaarController = TextEditingController();
final TextEditingController _captchaController = TextEditingController();
Future<void> verifyAadhaar() async {
final response = await ApiService.verifyAadhaar(
_aadhaarController.text,
_captchaController.text,
);
if (response != null) {
print('Verification Response: $response');
} else {
print('Failed to verify Aadhaar');
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Aadhaar Verification'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
children: [
TextField(
controller: _aadhaarController,
decoration: InputDecoration(labelText: 'Aadhaar Number'),
),
TextField(
controller: _captchaController,
decoration: InputDecoration(labelText: 'Captcha Code'),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: verifyAadhaar,
child: Text('Verify'),
),
],
),
),
);
}
}
Step 5: Running the App
A basic user interface (UI) including fields for your Aadhaar number and captcha code should appear. The application will perform an API connection to Setu's Aadhaar verification endpoint after you fill them out and click the Verify button, printing the verification response to the console.
Conclusion
Using Setu's OKYC API, this tutorial has demonstrated how to include Aadhaar verification into your Flutter application. This approach is easy to use and uncomplicated because it doesn't make use of any state management libraries.
If you are looking to integrating Aaddhar Card verfication into your Flutter Application. We can help you, with decade of experience in software development and integration our team will make sure all the integrations work perfect with your existing system. Visit us today!
FAQs
1. What is the importance of Aadhaar verification in fintech projects?
Aadhaar verification plays a critical role in ensuring compliance with KYC regulations, preventing fraud, and verifying user identities for services like digital banking, loan disbursement, and insurance approval.
2. How do I integrate Aadhaar verification into my Flutter app using Setu's API?
To integrate Aadhaar verification, set up your Flutter project, add dependencies, and create API calls using Setu’s OKYC API. Follow the step-by-step guide in the blog to create requests and handle responses for Aadhaar verification.
3. What are the prerequisites for implementing Aadhaar verification in Flutter?
You need a Flutter development environment, a Setu account with access to their Aadhaar verification API, and API credentials (x-client-id, x-client-secret, x-product-instance-id) to start the integration process.