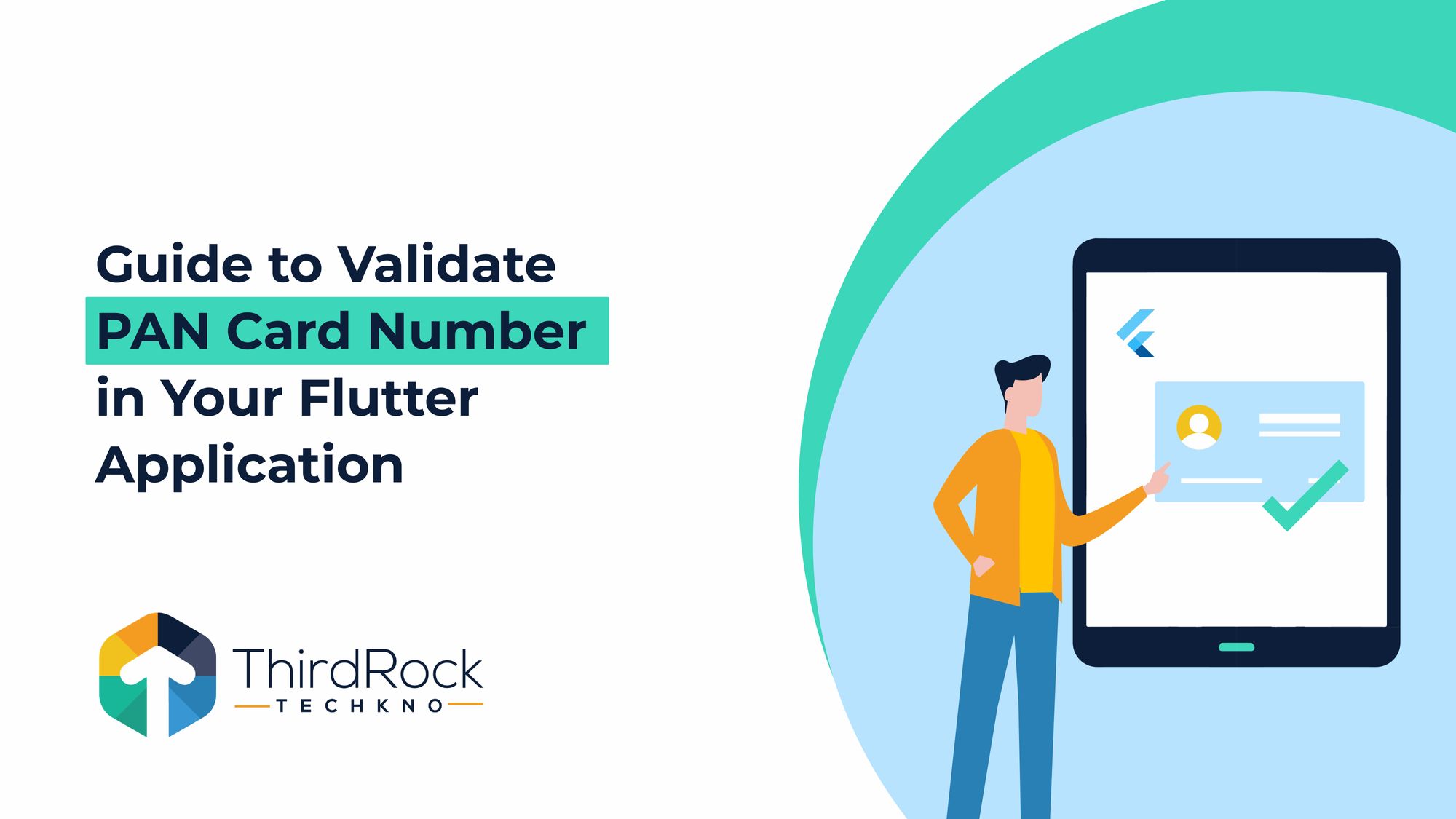
Table of Contents
Why PAN Card Verification is Important
Step 1: Configure Your Flutter Project
In this blog post, we will demonstrate how to use the Setu PAN verification API to integrate real-time PAN number verification in a Flutter application. We will cover creating a Flutter project, submitting queries to APIs, and managing responses in this tutorial.
Why PAN Card Verification is Important
PAN card verification is crucial in fintech applications, especially in banking, insurance, and financial services. Here's why:
- KYC Requirements: As part of the mandatory KYC process, verifying PAN cards ensures that users are authenticated and that financial transactions are legitimate.
- Legal Compliance: The Indian government mandates PAN card verification for transactions exceeding a certain threshold to avoid tax evasion and ensure regulatory compliance.
- Loan Approvals and Investment: In fintech, during the loan application process or when users are making significant financial investment, PAN card verification is essential to validate the user's identity.
What is Setu PAN API?
Setu PAN API allows you to use just one API to verify your customer’s PAN details. We directly connect with NSDL, to maintain the best uptimes.
Here’s a quick overview of the PAN API. Additionally, here are the URLs you would need for this API —
Headers — Contact Setu for providing the credentials required to successfully call Setu APIs. This contains:
- x-client-id
- x-client - secret
- x-product-instance-id
Verify PAN Card Number
Call this API to verify a PAN provided by your customer. A quick explanation of the request params—
- pan is the PAN value. It may belong to different categories like Person, Company, Trust, Government, Firm, etc.
- Consent indicates if you have collected consent from your customer. To get a successful verification, it must contain Y or y.
- reason is the explanation of why you are requesting for PAN from your customer. It should be explained in 20 characters or more.
Note: While the implementation of consent and reason cannot be enforced by Setu, we recommend collecting explicit consent from your customers and also explaining to your customers the reason why you are verifying their PAN.
While testing on Sandbox, you may use the following sample values
- Use ABCDE1234A for a valid PAN
- Use ABCDE1234B for an invalid PAN, i.e, a PAN number has been found but is invalid. A PAN is considered invalid by NSDL for different reasons.
For e.g, if it is a blacklisted one, or maybe because it is not linked to an Aadhaar card. - If you use any other values for pan, you will get a 404 PAN not found error.
Request Body
{
"data": {
"aadhaar_seeding_status": "LINKED", // optional
"category": "Individual",
"full_name": "John Doe"
},
"message": "PAN is valid",
"verification": "success",
"traceId": "1-6346a91a-620cf6cc4f68d2e30316881e"
}
Prerequisites
Make sure you have:
- A basic understanding of Flutter and Dart before you start.
- Flutter is installed on your system.
- A Setu account is required in order to use the PAN verification API. Here is where you may register. link
Step 1: Configure Your Flutter Project
If you don't already have a Flutter project, start by creating one:
flutter create pan_verification_app
cd pan_verification_app
Step 2: Add Dependencies
In your pubspec.yaml file, add the http, and get packages to handle HTTP requests and state management:
dependencies:
flutter:
sdk: flutter
http: ^0.14.0
get: ^4.6.5
Step 3: Create the API Service Class
To manage the API requests, create a new file called lib/services/api_service.dart:
import 'package:http/http.dart' as http;
import 'dart:convert';
class ApiService {
static const String apiUrl = 'https://dg-sandbox.setu.co/api/verify/pan';
static const String clientId = 'YOUR_CLIENT_ID';
static const String clientSecret = 'YOUR_CLIENT_SECRET';
static const String productInstanceId = 'YOUR_PRODUCT_INSTANCE_ID';
static const String bearerToken = 'YOUR_BEARER_TOKEN';
static Future<Map<String, dynamic>> verifyPan(String panNumber) async {
final response = await http.post(
Uri.parse(apiUrl),
headers: {
'x-client-id': clientId,
'x-client-secret': clientSecret,
'x-product-instance-id': productInstanceId,
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer $bearerToken',
},
body: json.encode({
'pan': panNumber,
'consent': 'Y',
'reason': 'Reason for verifying PAN set by the developer',
}),
);
if (response.statusCode == 200) {
return json.decode(response.body);
} else {
throw Exception('Failed to verify PAN: ${response.body}');
}
}
}
Step 4: Create the Controller
Create a new file lib/controllers/pan_controller.dart to handle the business logic:
import 'package:get/get.dart';
import '../services/api_service.dart';
class PanController extends GetxController {
var panNumber = ''.obs;
var result = ''.obs;
var isLoading = false.obs;
void verifyPan() async {
if (panNumber.value.isEmpty) {
result.value = 'Please enter a PAN number.';
return;
}
isLoading.value = true;
try {
final data = await ApiService.verifyPan(panNumber.value);
result.value = 'PAN Verification Successful: ${data['status']}';
} catch (e) {
result.value = 'PAN Verification Failed: $e';
} finally {
isLoading.value = false;
}
}
}
Step 5: Create the UI
In lib/main.dart, create the UI with GetX for state management:
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'controllers/pan_controller.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GetMaterialApp(
home: PanVerificationScreen(),
);
}
}
class PanVerificationScreen extends StatelessWidget {
final PanController _panController = Get.put(PanController());
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('PAN Verification'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
children: [
TextField(
onChanged: (value) => _panController.panNumber.value = value,
decoration: InputDecoration(
labelText: 'Enter PAN Number',
),
),
SizedBox(height: 20),
Obx(() => _panController.isLoading.value
? CircularProgressIndicator()
: ElevatedButton(
onPressed: _panController.verifyPan,
child: Text('Verify PAN'),
)),
SizedBox(height: 20),
Obx(() => Text(_panController.result.value)),
],
),
),
);
}
}
Step 6: Run the App
Use an emulator or connect your smartphone to launch the app, and run the command below:
flutter run.
Enter a valid PAN number in the text field and click the button to see the verification result.
Conclusion
In this article, we have discussed how to use Setu PAN verification API, GetX for state management, and splitting the API call into a distinct class to include real-time PAN number verification in a Flutter app.
By including error management, input validation, and an improved user interface, you may further improve this application. See the official Setu API documentation for further information. Official documentation
Note: In the blog, we are managing the state with GetX. Any alternative state management system that meets the requirements of your project is acceptable.
FAQs
- What is the importance of PAN card verification in fintech applications?
PAN card verification is important in fintech apps for verifying users' identities during processes such as KYC, loan applications, and large financial transactions. It ensures legal compliance and helps prevent tax evasion. - What is the Setu PAN API, and How does it work?
The Setu PAN API allows developers to verify PAN details by connecting with NSDL, the official PAN database. It ensures real-time verification, providing the user's name and the status of their PAN card. - What are the prerequisites for using the Setu PAN verification API?
You need basic Flutter and Dart knowledge, a Setu API account for API credentials (client ID, client secret, product instant ID), and the Flutter SDK installed on your machine to begin integration.